Understanding the Assistants API
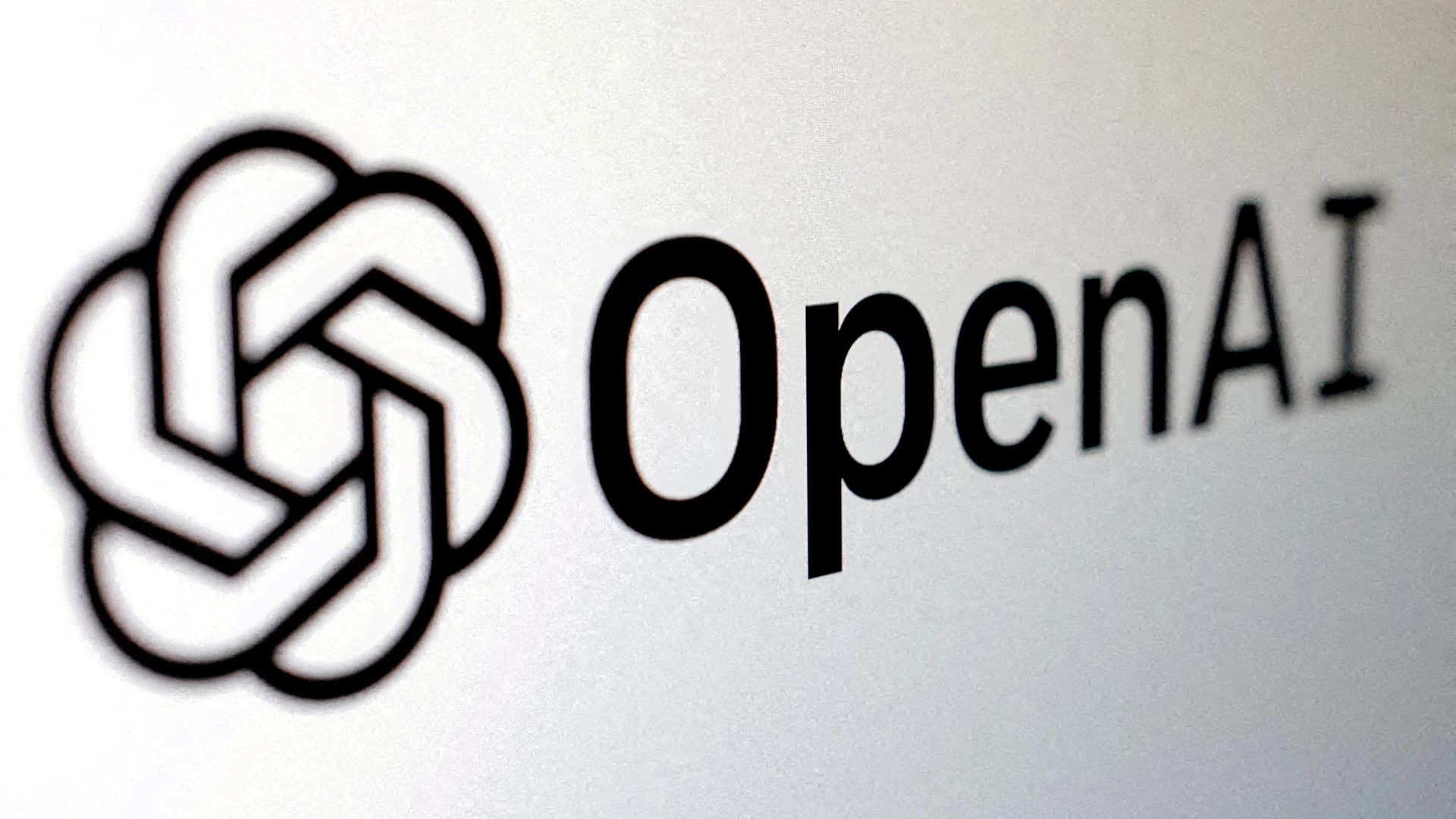
OpenAI’s latest innovation, the Assistants API (Beta), is a groundbreaking tool that enables developers to build highly customizable AI assistants capable of performing complex tasks. Whether you're a software developer or a machine learning engineer, this API allows you to integrate AI assistants directly into your applications.
It offers higher control than a custom GPT or a traditional OpenAI model API call.
Key Advantages
- Customization: Define assistant behavior with specific instructions.
- Tool Integration: Use tools like the Code Interpreter and File Search.
- Scalability: Manage multiple assistants and threads efficiently.
- Flexibility: Interact via the Playground or programmatically.
Core Concepts
- Assistant: The AI entity with instructions, tools, and a selected model.
- Thread: Represents a conversation session.
- Message: A user/assistant exchange.
- Run: Executes the assistant on a thread.
- Run Step: Details each step during a run (like tool usage).
Method 1: Creating Assistants via the Playground
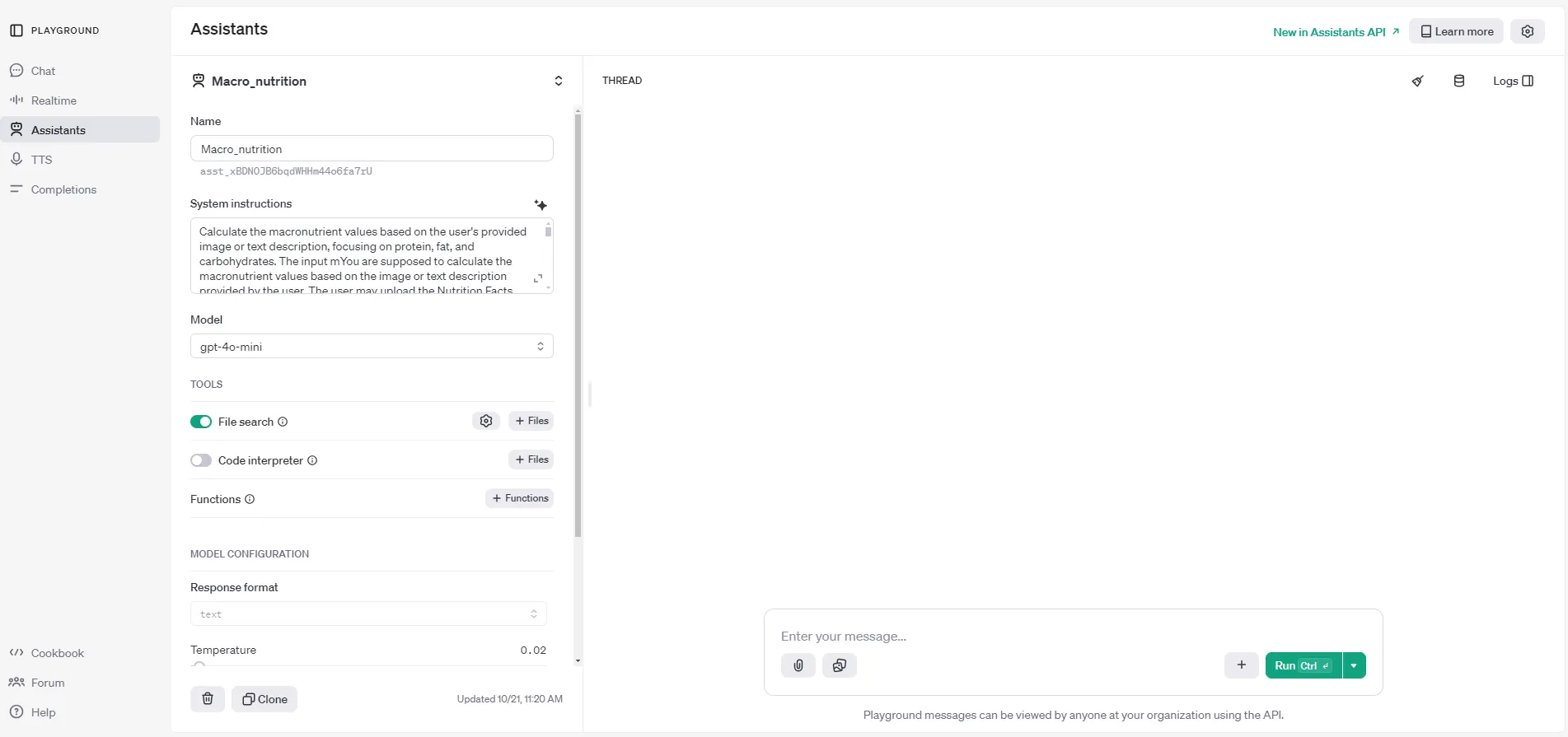
Step-by-Step
- Access the Playground: Log in to OpenAI Platform → Sidebar → Assistants.
- Create a New Assistant: Provide a name, description, and instructions.
Example: Calculate the Macronutrients: Fats, Proteins, and Carbohydrates based on user input. - Select the Model: Choose one (e.g.,
gpt-4o
) depending on your task. - Add Tools: Enable tools like the Code Interpreter.
- Configure Parameters: Tune values like
Temperature
,Top-p
, etc. - Test Interactions: Use the chat interface with messages like:
"I ate 200 Grams of Brown bread." - Iterate and Refine: Tweak and test based on response quality.
Method 2: Creating Assistants Using the API
For advanced users, the API allows deeper integration. Be sure to update to the latest SDK version to access Assistants API (Beta).
Step 1: Import OpenAI SDK
import openai
openai.api_key = 'YOUR_API_KEY'
Step 2: Create an Assistant
assistant = openai.Assistant.create(
model="gpt-4o",
name="Math Tutor",
instructions="Calculate the Macronutrients: Fats, Proteins and Carbohydrates based on user input.",
tools=[{"type": "code_interpreter"}],
temperature=1.0,
top_p=1.0,
response_format="auto"
)
Step 3: Create a Thread
thread = openai.Thread.create()
Step 4: Add Messages
message = openai.Message.create(
thread_id=thread.id,
role="user",
content="I ate 200 Grams of Brown bread."
)
Step 5: Run the Assistant
run = openai.Run.create(
thread_id=thread.id,
assistant_id=assistant.id
)
Sample Output
\begin{align*} \text{Calories} &= 250 \times 2 = 500 \\ \text{Carbohydrates} &= 43 \times 2 = 86 \\ \text{Protein} &= 9 \times 2 = 18 \\ \text{Fat} &= 3 \times 2 = 6 \end{align*}
Summary for 200 grams of brown bread:
- Calories: 500 kcal
- Carbohydrates: 86 g
- Protein: 18 g
- Fat: 6 g
Advanced Features & Best Practices
Run Steps
Track internal actions taken by the assistant:
run_steps = openai.RunStep.list(
thread_id=thread.id,
run_id=run.id
)
for step in run_steps.data:
print(step)
Token Management
run = openai.Run.create(
thread_id=thread.id,
assistant_id=assistant.id,
max_prompt_tokens=500,
max_completion_tokens=200
)
Structured Outputs
assistant = openai.Assistant.create(
model="gpt-4o",
instructions="Provide weather data.",
response_format={
"type": "json_schema",
"json_schema": {
"type": "object",
"properties": {
"temperature": {"type": "number"},
"condition": {"type": "string"}
},
"required": ["temperature", "condition"]
}
}
)
Integrating Custom Tools
assistant = openai.Assistant.create(
model="gpt-4o",
instructions="You can call functions to get stock prices.",
tools=[
{
"type": "function",
"name": "get_stock_price",
"description": "Retrieves the current stock price for a given symbol.",
"parameters": {
"type": "object",
"properties": {
"symbol": {"type": "string"}
},
"required": ["symbol"]
}
}
]
)
Resources
Whoossh, this was one of the longest articles I wrote. Let me know if I missed something. I’ve created a few assistants myself and will continue tinkering!